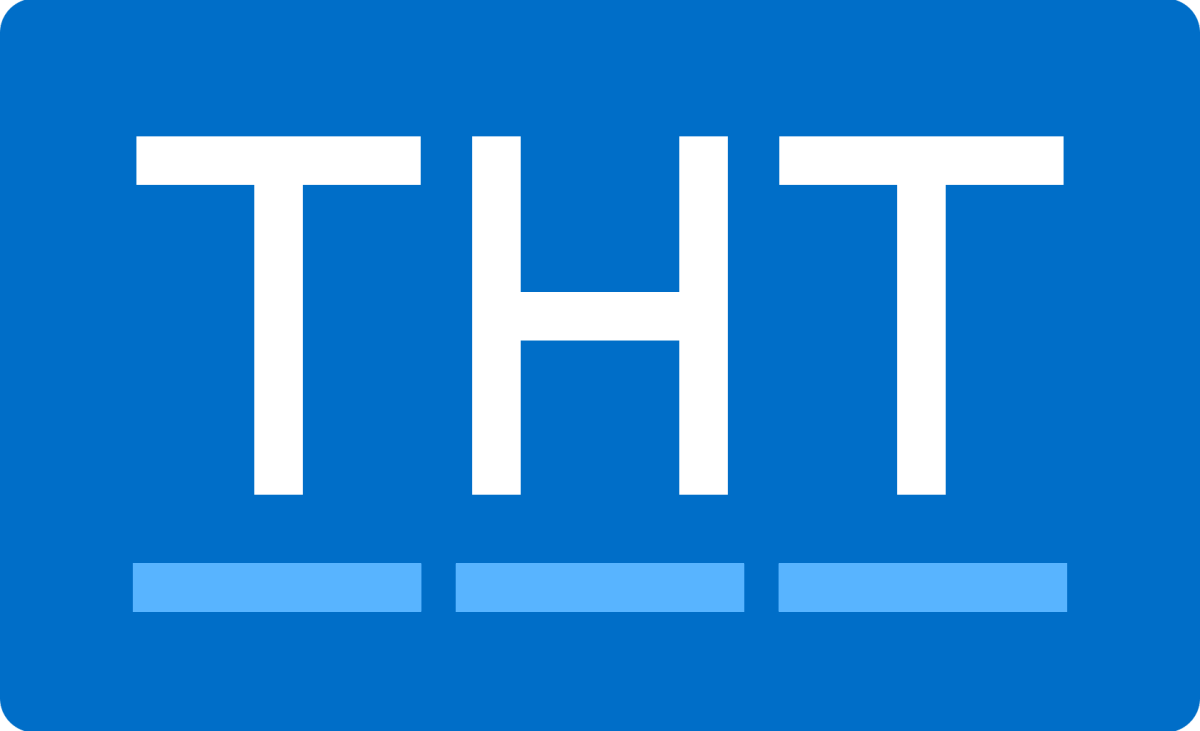
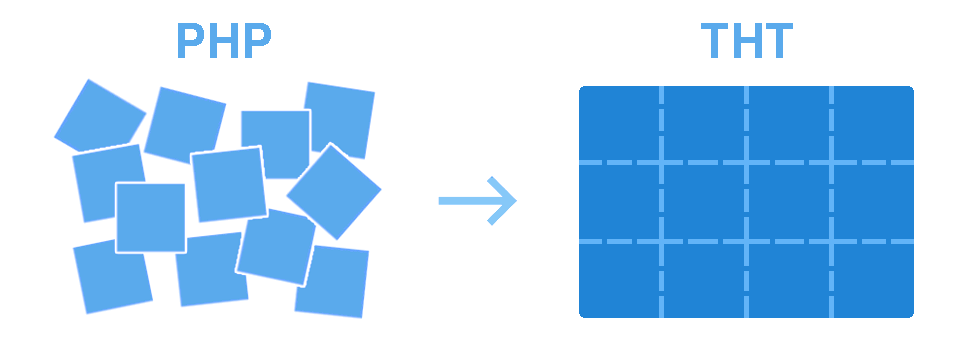
THT is a modern re-design of PHP, making it more secure & easier to use.
PHP | THT | |
---|---|---|
Easy to learn | ||
Simple workflow | ||
Cheap to host | ||
Fast with PHP 8+ | ||
Organized library | ||
Friendly error pages | ||
Built-in security | ||
Web framework |
A Quick Look
THT's streamlined syntax makes your code easier to
// THT code is 15% smaller than PHP. fun getCartTotal { $total = 0 $items = [ { id: 123, name: 'T-Shirt' } { id: 456, name: 'Teddy Bear' } ] foreach $items as $item { if !$item.name.contains('Not in Stock') { $total += Catalog.getPrice($item.id) } } return '$' ~ $total }
// THT code is 15% smaller than PHP. function getCartTotal() { $total = 0; $items = [ [ 'id' => 123, 'name' => 'T-Shirt' ], [ 'id' => 456, 'name' => 'Teddy Bear' ], ]; foreach ($items as $item) { if (!str_contains($item['name'], 'Not in Stock')) { $total += Catalog::getPrice($item['id']); } } return '$' . $total; }
Read More: Syntax Cheat Sheet | Full Language Tour
Key Language Features
PHP's massive library (2,000+ functions) is curated and organized into modules & classes.
// PHP in_array('needle', $hayStack) // THT $hayStack.contains('needle')
PHP arrays are split into distinct List and Map types.
// PHP [ 'name' => 'Toni', 'age' => 33 ] // THT { name: 'Toni', age: 33 }
Code is organized via modules, which are automatically namespaced and have controls for outside access.
// PHP require_once('Some/Module.php'); \Some\Module\doSomething(); // THT load('Some/Module') Module.doSomething()
String functions work with UTF-8 by default.
// PHP strlen('清潔な場所') //= 15 (✖ Wrong) // THT '清潔な場所'.length() //= 5 (✔ Correct)
A compile-time Format Checker helps keep your code clean and consistent.
// Valid PHP if($Some_VAR==$DBrow [ 'column' ]){ $A =$B *3; } // THT if $someVar == $dbRow['column'] { $a = $b * 3 }
The error pages are easy to read, and include full stack traces, suggested fixes, and links to documentation.
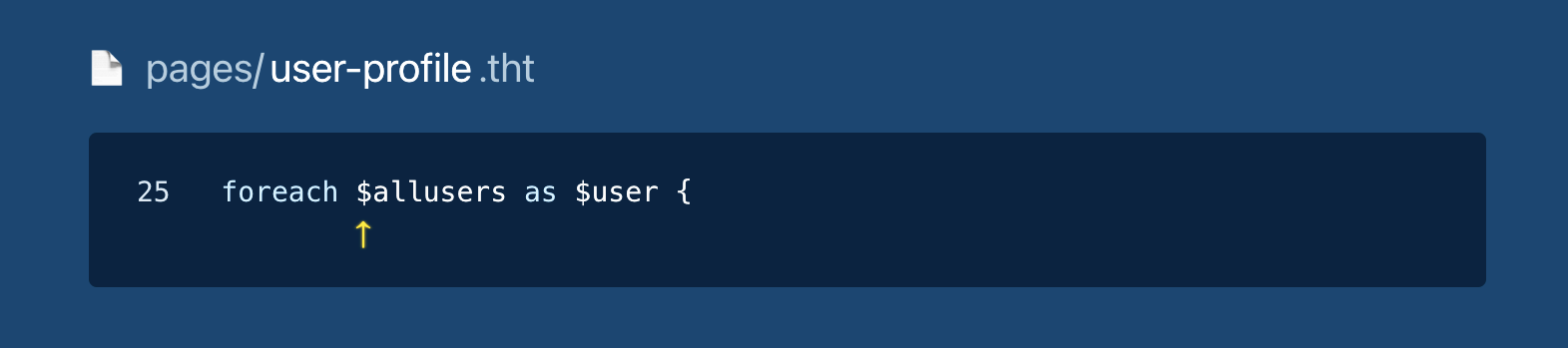
Read More: How THT Compares to PHP
Web Framework
The URL router makes it easy to give every page a clean URL.
// PHP /blog/posts.php?postId=1234 // THT /blog/posts/1234/my-favorite-things
Template functions let you cleanly organize your view-level code in a way that is both flexible and fast.
Templates support HTML-C shortcuts, and automatically escape variables to prevent XSS attacks.
// PHP function messagesHtml($messages) { ?> <div class="messages"> <?php foreach ($messages as $m) { ?> <div class="message"><?= $m['subject'] ?></div> <?php } ?> </div> <?php } // THT tem messagesHtml($messages) { <.messages> --- foreach $messages as $m { <.message> {{ $m.subject }} --- } </> }
THT validates all user input by default, including secure file uploads.
// PHP - No validation (✖ Unsafe) $userId = $_GET['userId']; // THT - Validate as a positive (i)nteger $userId = Input.get('userId', 'i')
The Form module makes it easier to build mobile-friendly forms. Input fields are validated on both the client (for usability) and the server (for security).
The Session module is built with secure defaults and has methods for common tasks like setting fire-and-forget flash data.
$cartKey = 'shoppingCart' Session.addToList($cartKey, 'T-Shirt') Session.addToList($cartKey, 'Teddy Bear') Session.get($cartKey) //= ['T-Shirt', 'Teddy Bear']
The Database module has convenience methods for CRUD operations.
It uses SQL TypeStrings to prevent injection attacks.
$query = sql'select * from posts where userId = {}' $query.fill(123) Db.selectRows($query)
The Email module makes it easy to send email (HTML or plaintext) through a third-party email sender.
Email.send({ from: 'you@yoursite.com' to: 'user@mail.com' subject: 'Welcome!' body: ''' Thanks for joining! ''' })
THT comes with an optional lightweight CSS framework with reset styles, a responsive grid system, and SVG icons.
THT introduces Litemark, a Markdown variant that supports different authoring modes (e.g. user comments vs documentation) and the creation of custom tags.
# Heading Bullets: - Here is __italic text__ - Here is **bold text** - Here is an [image /some/image.png] - Here is a [/some/url | link to another page]
Built-In Security
THT is designed from the ground up with security best practices and secure defaults, protecting your app against the most common security risks.
Unlike other programming languages that rely on third-party frameworks and manual effort for security, THT builds safeguards into the language itself so that fewer vulnerabilities can slip through the cracks.
TypeStrings
TypeStrings are compile-time templates that are used to prevent injection attacks, which is one of the top security vulnerabilities for web apps.
// SQL TypeString $q = sql'select name from users where id = {}' ^^^ // Fill in the placeholder {} $q.fill(123) // Other types include 'url', 'cmd', etc. $u = url'/users?sort={}' ^^^
Other Safeguards
- File functions can’t be used with external (potentially dangerous) URLs.
- Post requests are protected by an auto-generated CSRF token.
- And many more...
Read More: All Security Enhancements
Performance
THT runs on PHP 8, which has been significantly optimized for speed, and can handle thousands of requests per second.
In a basic speed test, THT runs 14x faster than the Laravel framework.
Framework | Requests/sec | (More = Faster) |
---|---|---|
Laravel | 352 | |
THT | 5,188 |
On a MacBook Pro, the core THT unit tests (1,200+ tests) run in about 40 milliseconds.
THT also has built-in performance tools:
- The Perf Panel is an easy way to profile your overall page speed.
- The Cache module can help you minimize performance bottlenecks.
- Images and assets are automatically optimized, reducing sizes by up to 70%.
How It Works
Same Simple Workflow
THT keeps the same easy “edit & refresh” workflow of PHP.
- Files are automatically transpiled to PHP on the first request, then cached for performance.
- There are no build steps or server restarts to disrupt your flow.
Under The Hood
- The transpiled code is compatible with PHP 8.3.
- The transpiler itself is written in 100% PHP with no dependencies.
- It runs on any platform that PHP runs on, including shared web hosts.
PHP Integration
You can call PHP functions from THT via the PHP Interface.
Get Started
THT is still in Beta, so it’s currently only recommended for early adopters.
You can also follow THT's ongoing progress here: